Automated Service Ticket Analysis System
turning maintenance records into structured actionable data
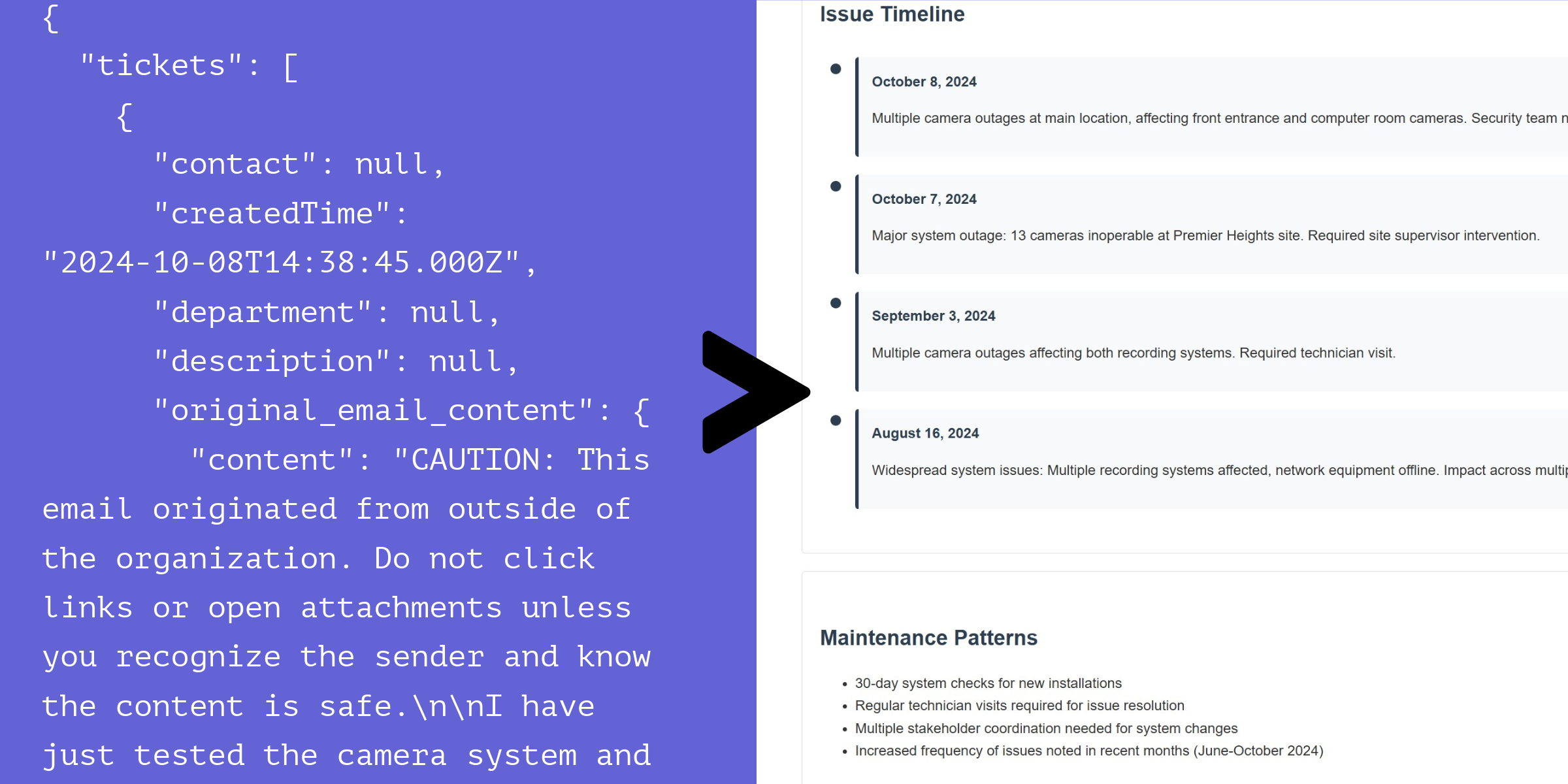
Client
Developed for a physical security company to enhance user experience and streamline operations.
Date
Completed in October 2023
My Role
Project Overview
This project demonstrates my expertise in integrating Large Language Models (LLMs) into existing business applications to enhance operational efficiency. By developing an automated service ticket analysis system that leverages llama 3.2, I transformed a manual ticket review process into a streamlined, intelligent workflow. The system processes unstructured maintenance tickets into actionable data, with careful attention to maintaining data fidelity through iterative structuring. Through sophisticated prompt engineering and AI integration techniques, the system consistently extracts critical information from varied ticket formats, enabling automated pattern recognition and trend analysis that was previously impossible with manual processing. The implementation showcases my ability to bridge traditional business processes with cutting-edge AI capabilities, resulting in meaningful improvements to service quality and operational efficiency. This project highlights my skill in identifying opportunities for AI enhancement in established workflows and successfully implementing solutions that drive tangible business value.
Key Technical Achievements
-
Development of an advanced prompt engineering system that maintains high fidelity data extraction across varied ticket formats
-
Implementation of a resilient API integration layer with intelligent retry mechanisms and rate limiting protection
-
Creation of a flexible JSON-based data structure that accommodates varied ticket information while maintaining consistency
-
Integration of sophisticated error handling and validation systems to ensure reliable operation
-
Development of automated pattern recognition and trend analysis capabilities
Innovative AI Integration Approaches
-
Iterative prompt refinement methodology ensuring consistent extraction of critical information
-
Sophisticated data structuring techniques that maintain fidelity while enabling automated analysis
-
Careful attention to rate limiting and API efficiency through intelligent request management
-
Implementation of automated trend analysis and pattern recognition systems
-
Integration with existing business workflows while minimizing disruption
Implementation Impact
This implementation demonstrates not only technical proficiency in AI integration but also strategic thinking in business process optimization. The system’s ability to transform manual processes into automated workflows while maintaining data quality showcases both practical implementation skills and business acumen. The project serves as a prime example of identifying opportunities for AI enhancement in established workflows and successfully implementing solutions that drive tangible business value.
Project Success and Value
The success of this project highlights the potential for AI integration in traditional business processes and demonstrates the ability to bridge the gap between cutting-edge technology and practical business needs. The implementation has resulted in measurable improvements in operational efficiency and service quality, proving the value of thoughtful AI integration in business operations.
Prompt: Stage 1
def process_with_llama(ticket, max_retries=5, initial_wait=1):
client = llama.Client()prompt = f”””
You are an AI assistant analyzing a customer support ticket. Your task is to extract key information from the ticket, including the date, main issue, affected equipment, and to classify the ticket. Please follow these guidelines:– Answer only based on the information provided in the ticket content.
– If you can’t find specific information, indicate that it’s not available.
– Prioritize high fidelity extraction over strict formatting.
– Include all relevant details, even if they don’t fit neatly into predefined categories.
– Do not make assumptions or add information not present in the ticket content.Here’s the extracted information from the ticket:
{json.dumps(ticket, indent=2)}Please extract and provide the following information:
1. Ticket Date: Provide the date of the ticket. If multiple dates are mentioned, provide the earliest date in the ticket content.
2. Ticket Classification: Classify the ticket into one of the following categories:
– ‘footage request’: If the ticket is about requesting video footage.
– ‘equipment malfunction’: If the ticket is about any equipment not working properly.
– ‘credential update’: If the ticket is about updating access credentials, passwords, or similar.
– ‘other general request’: If the ticket doesn’t fit into the above categories.3. Main Issue: Provide a brief summary of the main issue described in this ticket. Include any relevant context or urgency mentioned.
4. Affected Equipment: List all equipment mentioned as having problems. Include as much detail as possible:
– For DVRs/NVRs: List each one separately and include any associated cameras.
– For Cameras: List all mentioned cameras, grouping them with their associated DVR/NVR if that information is available.
– For other equipment (e.g., Dishes, FOBS): List these separately with any associated details.5. Additional Context: Include any other relevant information from the ticket that provides context about the issue, such as:
– Any mentioned troubleshooting steps
– Scheduled maintenance or repair times
– Customer contact information
– Any other details that seem important for understanding the full context of the ticketFormat your response as a JSON object. The structure should be flexible to accommodate all extracted information, but try to group related information together. Here’s a suggested structure, but feel free to modify it as needed to capture all relevant details:
{{
“ticket_date”: “Extracted date”,
“ticket_classification”: “One of the four categories”,
“main_issue”: “Summary of the main issue”,
“affected_equipment”: {{
“dvr_nvr”: [
{{
“name”: “DVR/NVR identifier”,
“details”: “Any additional details about this DVR/NVR”,
“associated_cameras”: [“List of associated cameras”]
}}
],
“cameras”: [“List of all mentioned cameras”],
“other_equipment”: [
{{
“type”: “Equipment type”,
“details”: “Details about this equipment”
}}
]
}},
“additional_context”: {{
“troubleshooting”: [“Any mentioned troubleshooting steps”],
“scheduled_actions”: [“Any scheduled maintenance or repairs”],
“customer_info”: {{
“name”: “Customer name if mentioned”,
“contact”: “Contact information if available”
}},
“other_details”: [“Any other relevant information”]
}}
}}Remember, the goal is high fidelity extraction. If you find information that doesn’t fit neatly into this structure, include it anyway in a way that makes sense. Use null for any fields where information is not available.
“””